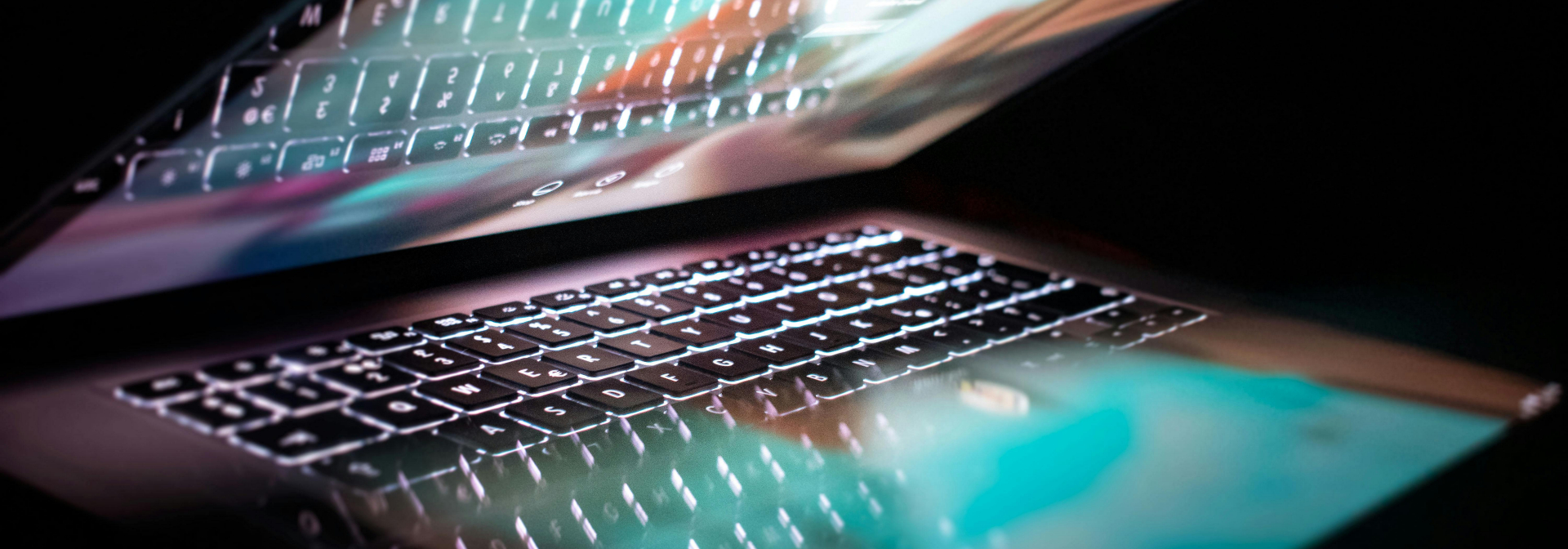
Web development is a dynamic and ever-evolving field that plays a pivotal role in shaping the digital landscape. It encompasses a vast array of technologies, languages, and frameworks, all aimed at creating, designing, and maintaining websites and web applications.
At the heart of web development lies HTML (Hypertext Markup Language) and CSS (Cascading Style Sheets). These two languages form the foundational building blocks of the web.
HTML provides the structure for web pages by defining the elements that organize content. It uses tags enclosed in angle brackets to mark up content, making it possible for browsers to render the page. HTML elements include headings, paragraphs, images, links, and more.
While HTML defines the structure, CSS takes care of the presentation. CSS is used to style HTML elements, determining their size, color, position, and layout. It allows developers to create visually appealing web pages.
Full stack development is a holistic approach that involves working with the full stack of the involved technology layers. Full stack developers work on both the client side (front-end) and server side (back-end) of web applications. Full stack developers are proficient in a range of technologies and can tackle every aspect of a project.
Back-end development focuses on server-side logic, databases, and the application's core functionality. Technologies like Java, Python, and Node.js are commonly used for back-end development.
Front-end development concentrates on the user interface and user experience. It involves creating interactive and visually appealing web pages using HTML, CSS, and JavaScript.
Databases are central to web applications for storing, retrieving, and managing data. Relational databases like MySQL, PostgreSQL, and NoSQL databases like MongoDB are widely used.
Full stack developers often work with frameworks and libraries that streamline development. For example, the Spring framework in Java simplifies back-end development, while React.js and Angular enhance front-end development.
Spring is a powerful framework for building enterprise-level Java applications. It simplifies the development of complex, robust, and scalable applications.
Spring implements Inversion of Control (IoC), which allows developers to define the relationships between components and manage their lifecycle. This reduces complexity and enhances modularity.
Spring supports Aspect-Oriented Programming (AOP), allowing developers to modularize cross-cutting concerns like logging, security, and transactions. AOP separates concerns in large-scale applications.
Spring Boot, an extension of the Spring Framework, simplifies the development of production-ready applications. It offers auto-configuration, embedded web servers, and starter templates for various application types.
JavaScript is a versatile scripting language that enhances the interactivity and functionality of web pages. It is a fundamental component of modern web development.
JavaScript is at the core of front-end development. It enables the creation of dynamic and interactive user interfaces. Libraries and frameworks like jQuery, Angular, and React.js are commonly used for front-end development.
Node.js, a server-side JavaScript runtime, has gained popularity for back-end development. It allows developers to use JavaScript on the server side, streamlining the development process.
JavaScript boasts a rich ecosystem of libraries and frameworks.
A fast, small, and feature-rich JavaScript library that simplifies DOM manipulation and event handling.
A comprehensive front-end framework for building complex web applications with powerful features like two-way data binding and dependency injection.
A JavaScript library for building user interfaces, known for its component-based architecture and efficient rendering.
Learn more about JavaScript here.
Single-Page Applications (SPAs) are web applications that load a single HTML page and dynamically update content as the user interacts with the application. They provide a seamless, app-like user experience.
SPAs have several key features. Below we list them.
SPAs load only the necessary content, reducing initial loading times and providing a smoother user experience.
Content changes occur without full page reloads, creating seamless transitions.
SPAs manage application state, making it easier to handle user interactions and provide a responsive experience.
SPAs often have complex user interfaces with interactive components and dynamic data retrieval.
Angular is a front-end framework developed by Google that simplifies the development of dynamic, single-page web applications.
Angular offers several key features:
Angular applications are built using components, which encapsulate the application's logic, data, and user interface.
Angular uses two-way data binding, meaning changes in the user interface are immediately reflected in the underlying data model, and vice versa.
Angular's dependency injection system helps manage the application's components and their dependencies.
Directives are custom HTML attributes used to create dynamic, reusable components within the application.
React.js, developed by Facebook, is a JavaScript library for building user interfaces. It is renowned for its component-based architecture and efficient rendering.
React.js applications are composed of components. Each component encapsulates a part of the user interface and its behavior. Components can be reused, making it easy to build complex user interfaces.
React.js uses a Virtual DOM to optimize rendering. It updates only the parts of the actual DOM that have changed, improving performance and ensuring a responsive user experience.
React.js follows a unidirectional data flow, ensuring that data is passed down from parent to child components. This simplifies data management and helps prevent unexpected side effects.
Node.js is a server-side JavaScript runtime that has gained popularity for building fast and scalable network applications. It is known for its asynchronous, event-driven architecture.
Node.js excels at handling many concurrent connections with minimal overhead. Its non-blocking I/O operations allow it to efficiently manage multiple tasks, making it a solid choice for real-time applications and APIs.
Node.js has a vast package ecosystem thanks to npm (Node Package Manager). Developers can easily find and use open-source libraries to extend Node.js's capabilities.
Node.js is commonly used for server-side development. It can handle HTTP requests, serve web pages, and create RESTful APIs.
In addition to the core technologies and concepts discussed above, web development encompasses a wide array of other important elements.
Web servers like Apache, Nginx, and Microsoft Internet Information Services (IIS) are responsible for serving web content to clients (browsers). They play a critical role in handling HTTP requests and responses.
While databases were mentioned in the context of full stack development, it's worth noting that there are various types of databases, including relational databases (e.g., MySQL, PostgreSQL) and NoSQL databases (e.g., MongoDB, Cassandra). Choosing the right database for a project is a crucial decision in web development.
Understanding web hosting services is important. Shared hosting, virtual private servers (VPS), cloud hosting, and dedicated servers all have their unique advantages and use cases. Choosing the appropriate hosting solution is vital for web application deployment.
CMS platforms like WordPress, Drupal, and Joomla are widely used for creating and managing websites and web applications. These systems simplify content creation and management.
Web developers must be well-versed in web security principles to protect against common threats like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Technologies like HTTPS and security headers are essential for safeguarding web applications.
Representational State Transfer (REST) is an architectural style for designing networked applications. RESTful APIs are essential for enabling communication between different parts of a web application and external services.
Ensuring that web content is accessible (for example, for people with disabilities or those using a slow internet connection) is crucial. Web Content Accessibility Guidelines (WCAG) provide standards and guidelines for creating accessible websites.
Techniques like image optimization, code minification, and using Content Delivery Networks (CDNs) are vital for improving website performance and reducing page load times.
Adhering to web standards and validating HTML and CSS code helps ensure cross-browser compatibility and consistency. The World Wide Web Consortium (W3C) provides these standards.
Analyzing user behavior and website performance is crucial for making data-driven decisions. Tools like Google Analytics and Adobe Analytics are commonly used for web analytics.
PWAs are web applications that offer a native app-like experience in the browser. They can work offline, send push notifications, and provide an engaging user experience.
Web Assembly is a binary instruction format that enables high-performance execution of code on web browsers. It can be used for computationally intensive tasks and is supported by all major browsers.
WebSockets enable real-time, bidirectional communication between a client and a server. They are crucial for applications that require live updates, such as chat applications and online gaming.
Technologies like Docker and Kubernetes are used for containerizing web applications and managing them at scale.
In addition to the information laid out above, there are a handful of important terms you should also know:
Want to learn more about web development? Additional information can be found in the blog posts and books listed below.
Learn more computing from our official Learning Center.
And to continue learning even more about web development, sign up for our weekly blog recap here.