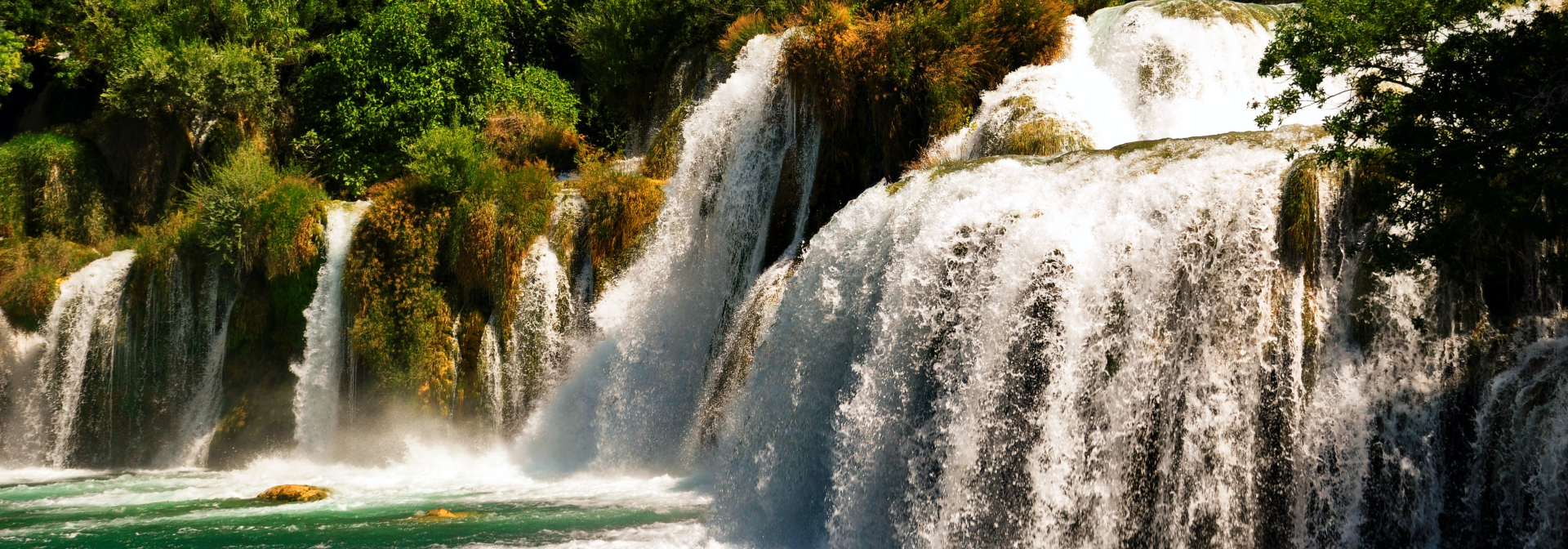
Java is a robust, versatile, and widely used programming language known for its platform independence, security features, and extensive ecosystem. It has made significant contributions to the world of software development, powering applications ranging from mobile apps to large-scale enterprise systems.
Java was created by James Gosling at Sun Microsystems in the mid-1990s, with the primary goal of developing a language suitable for embedded systems. Over the years, it has evolved into a versatile language with various applications. Below is a list of the key features of Java.
One of Java's most significant advantages is its platform independence. Java code is compiled into an intermediate representation called bytecode, which can run on any system with a Java Virtual Machine (JVM). This "write once, run anywhere" principle has made Java the go-to language for cross-platform development.
Java is a strongly typed language, meaning that variables must have specific data types, and type safety is enforced by the compiler. It is also object-oriented, which encourages the use of classes and objects to structure code. This makes Java code more organized and maintainable.
Java is known for its emphasis on security. The JVM includes features like bytecode verification, which helps prevent common security vulnerabilities. Additionally, Java manages memory automatically through garbage collection, reducing the likelihood of memory-related bugs.
Java includes a vast standard library that covers a wide range of tasks, from I/O operations and network communication to data manipulation and multithreading.
The Spring Framework is a popular choice for building enterprise applications in Java. It provides a comprehensive ecosystem for developing robust, scalable, and maintainable applications. Key features of the Spring Framework include the following.
Spring implements the Inversion of Control (IoC) principle, also known as the Dependency Injection (DI) pattern. This allows developers to define the relationships between components and manage their lifecycle. IoC simplifies application development and enhances modularity. A Spring Bean is an fundamental object managed by the Spring IoC container, configured to fulfill specific roles in a Spring application, with its lifecycle managed by the container.
Spring supports Aspect-Oriented Programming (AOP), which enables developers to modularize cross-cutting concerns, such as logging, security, and transactions. AOP simplifies the separation of concerns in large-scale applications.
Spring is widely used for web development, ranging from simple RESTful APIs to complex web applications. It provides the tools and features necessary to build robust and scalable web solutions. Here's how Spring is utilized in web development.
Spring MVC (Model-View-Controller) is a key component of Spring for web development. It allows developers to create web controllers to handle HTTP requests and produce responses.
Spring simplifies the creation of RESTful APIs through the use of annotations like @RestController and @RequestMapping. This allows developers to build APIs for a wide range of applications, from mobile apps to microservices.
Security is a critical aspect of web development. Spring provides tools and features for implementing authentication, authorization, and protection against common security threats. The Spring Security module is widely used for securing web applications.
Spring provides seamless integration with databases through the use of the Spring Data module and various data source configurations. It supports both SQL and NoSQL databases, making it suitable for a wide range of web applications.
Spring Boot is an extension of the Spring Framework that simplifies the process of building production-ready applications. It provides auto-configuration, an embedded web server, and a range of starter templates for various application types.
Spring Boot takes care of setting up the application context, configuring necessary components, and starting the embedded web server.
This is a game-changer in Java web development, as it offers a simplified and streamlined approach to building web applications. Key features of Spring Boot include the following.
Spring Boot provides auto-configuration, which automatically configures various components based on the project's dependencies. This reduces the need for extensive configuration files and setup, making development more efficient.
Spring Boot includes embedded web servers like Tomcat, Jetty, and Undertow, allowing developers to run applications as standalone executables. This eliminates the need to deploy applications on external servers, simplifying deployment and maintenance.
Spring Boot comes with production-ready features, such as health checks, metrics, and monitoring. This ensures that applications are equipped to handle production environments without additional configuration.
Spring Boot offers starter templates for various project types, including web applications, microservices, and data processing. These templates jumpstart development by providing a pre-configured project structure.
Microservices architecture is a popular approach for building scalable and maintainable applications. Spring Boot is frequently used for developing microservices, thanks to its lightweight and modular design. Here's how Spring Boot is employed in microservices.
Each microservice in a Spring Boot-based microservices architecture is typically a standalone Spring Boot application. These services can handle specific tasks or functionality, such as user authentication, data processing, or notifications.
Microservices often need to communicate with each other. Spring Boot supports various communication methods, including RESTful APIs, message queues, and gRPC. This allows microservices to work together to provide a complete application.
To manage and deploy microservices at scale, technologies like Docker and Kubernetes are often used. Spring Boot applications can be containerized and orchestrated to ensure high availability, scalability, and fault tolerance.
Java is a popular choice for implementing algorithms and data structures due to its strong typing, excellent performance, and vast ecosystem of libraries and tools. Here are some of the ways Java is employed in algorithm development.
Java provides a comprehensive set of data structures, including arrays, lists, stacks, queues, trees, and hash tables. These structures are fundamental for implementing various algorithms efficiently.
Java's standard library includes efficient sorting algorithms, such as the quicksort and mergesort implementations in the java.util.Collections class. These algorithms are essential for sorting data in various applications.
Java is commonly used for graph algorithms like depth-first search (DFS), breadth-first search (BFS), and shortest path algorithms. Libraries like JGraphT provide a rich set of tools for graph-related tasks.
Java has a long history in mobile and desktop app development and remains one of the primary languages for creating Android applications. Android Studio, the official integrated development environment for Android, supports Java alongside Kotlin, another popular language for Android development.
Android Studio is the go-to tool for developing Android applications in Java. It provides a rich set of features for designing user interfaces, coding application logic, and testing on emulated or physical Android devices.
The Android Software Development Kit (SDK) provides libraries, tools, and APIs for developing Android applications. Java developers can leverage the Android SDK to build a wide variety of apps, from simple utilities to complex games.
In addition to Android app development, Java is also used for building desktop applications. JavaFX is a framework that allows developers to create rich and interactive graphical user interfaces for applications running on various platforms, including Windows, macOS, and Linux.
Java is also used in scientific computing for tasks like data analysis, simulations, and visualization. While Python is often the first choice for such tasks, Java offers advantages in certain scenarios.
Java's strict typing and strong performance make it suitable for computationally intensive tasks. For certain scientific simulations, Java's execution speed can be advantageous.
Java supports parallelism and multithreading, allowing for efficient use of multiple processor cores. This is essential for scientific computing tasks that require parallel processing.
Java provides libraries for data visualization, such as JavaFX and JFreeChart, which are valuable for presenting scientific results and creating interactive data visualizations.
For large-scale enterprise applications, Java EE, now known as Jakarta EE, offers a suite of specifications and APIs for building robust and secure enterprise-level software. This includes features like servlets, JSP, JMS (Java Message Service), and EJB (Enterprise JavaBeans).
In addition to the information laid out above, there are a handful of important terms you should also know:
Want to learn more about Java? Additional information can be found in the blog posts and books listed below.
Learn more computing from our official Learning Center.
And to continue learning even more about Java, sign up for our weekly blog recap here.