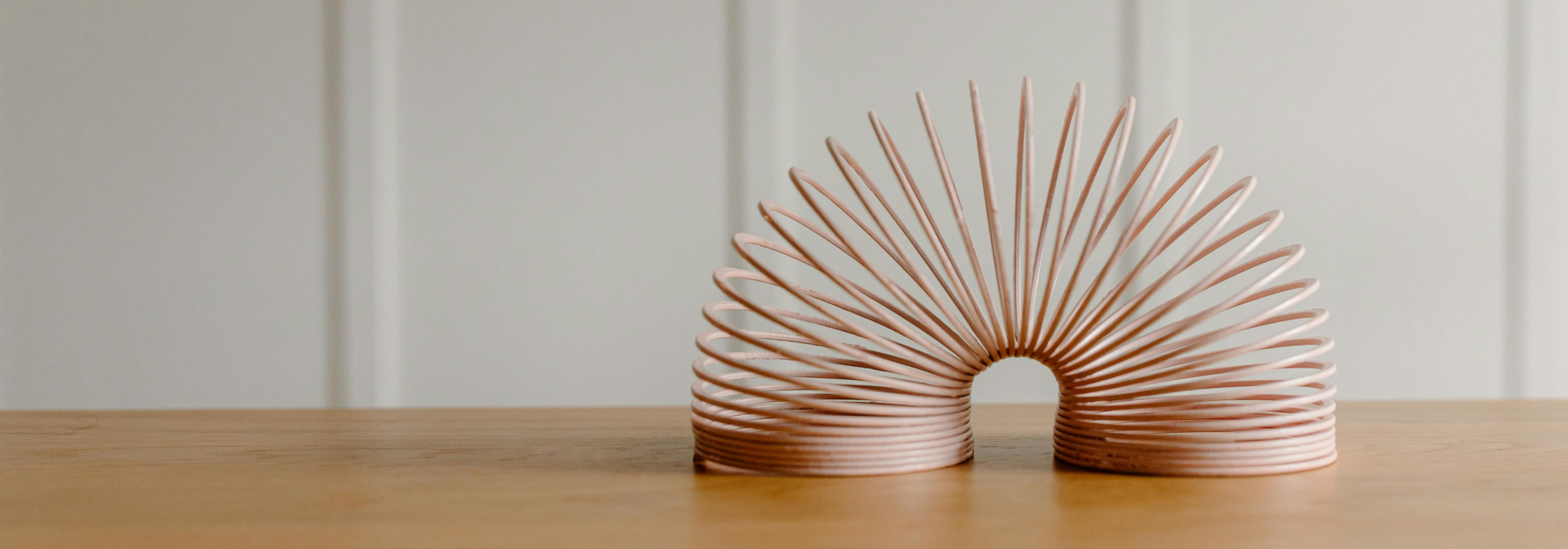
JavaScript is a versatile and widely used programming language that plays a fundamental role in web development. It empowers developers to create interactive and dynamic web applications.
JavaScript is a high-level, interpreted, and dynamically typed scripting language primarily used for client-side web development. Understanding its foundational concepts is crucial for anyone entering the world of web development. Below we list its basic concepts.
JavaScript's syntax is similar to many other programming languages, making it accessible to developers with various backgrounds. It uses variables, data types, and functions to manipulate data and perform actions.
One of the primary use cases for JavaScript on the frontend is interacting with the Document Object Model (DOM). The DOM represents the structured content of a web page, and JavaScript enables developers to dynamically modify it. This allows for activities like adding, deleting, or altering HTML elements and content in response to user interactions.
JavaScript can capture and respond to user events, such as clicks, key presses, and mouse movements. Event listeners facilitate the creation of interactive web pages. JavaScript is therefore used in conjunction with HTML and CSS when developing for the web.
Modern web applications often involve tasks that take time to complete, such as fetching data from a server. JavaScript supports asynchronous programming through mechanisms like callbacks, Promises, and the async/await syntax. This allows developers to initiate operations and continue executing code without waiting for the operation to finish. This is good, for example, for creating web tables with hundreds or thousands of rows and columns of data.
JavaScript libraries are collections of pre-written code that simplify common web development tasks. They streamline the development process, reduce development time, and enhance productivity. Let's explore some popular JavaScript libraries and their use cases.
jQuery is a fast, small, and feature-rich library that simplifies HTML document traversal, event handling, and animation. It was extremely popular in the early 2010s and is still used in some legacy projects. jQuery eases the creation of dynamic and interactive web applications.
For example, jQuery allows you to select elements and perform actions on them with concise syntax.
D3.js (Data-Driven Documents) is a powerful library for creating data visualizations on the web. It enables developers to bind data to HTML, SVG, and CSS, creating compelling charts and graphs. D3.js provides extensive capabilities for creating custom data visualizations, and it is widely used for data-driven storytelling on the web.
Lodash provides utility functions for common programming tasks, such as iterating over arrays, manipulating objects, and working with strings. It enhances code readability and performance. Lodash's functions are designed to be consistent and work across various JavaScript environments.
Handling dates and times in JavaScript can be challenging due to the native Date object's limitations. Moment.js is a library that makes working with dates and times more straightforward. It allows parsing, validating, manipulating, and formatting dates and times with ease.
JavaScript frameworks are larger, more comprehensive collections of libraries and tools. They offer a structured way to build web applications and promote best practices. Let's explore some of the most prominent JavaScript frameworks and their key features.
Developed by Facebook, React is a popular JavaScript library for building user interfaces. React follows a component-based architecture, which means that UIs are built by composing reusable components. One of the standout features of React is the virtual DOM, which optimizes performance by minimizing the number of actual DOM updates.
Angular is an open-source framework developed by Google. It provides a comprehensive platform for building web, mobile, and desktop applications. Angular emphasizes declarative templates and dependency injection, which makes it suitable for large and complex applications.
cVue.js is a progressive framework for building user interfaces. It's known for its simplicity and ease of integration into existing projects. Vue.js also uses a virtual DOM and follows a component-based structure, similar to React.
While not a front-end framework, Node.js is worth mentioning in the context of JavaScript. Node.js is a runtime environment that allows developers to run JavaScript on the server-side. It's commonly used for building scalable network applications, such as web servers and APIs. Node.js's non-blocking, event-driven architecture makes it well-suited for handling concurrent connections.
JavaScript developers often use package managers to manage project dependencies and streamline project setup. Two of the most widely used package managers in the JavaScript ecosystem are npm (Node Package Manager) and Yarn.
npm is the default package manager for Node.js. It simplifies the installation of libraries, frameworks, and other dependencies. Developers can specify project dependencies in a package.json file, making it easy to share code and ensure consistency across projects.
Yarn is another popular package manager developed by Facebook. It offers performance improvements over npm and is fully compatible with npm packages. Yarn is known for its speed and security features, making it a preferred choice for many developers.
JavaScript module systems play a crucial role in organizing and structuring code in large-scale applications. They provide a way to encapsulate code into reusable and independent units, promoting modularity and maintainability. Below lists some of the more common JavaScript module systems, highlighting their features and usage.
CommonJS is a module system used primarily in server-side environments, notably with Node.js. It relies on the require function to import modules and the module.exports object to export functionalities. This system allows developers to create modular code by breaking down applications into smaller, manageable pieces.
With the advent of ECMAScript 2015 (ES6), JavaScript introduced native support for modules. ES6 modules provide a standardized syntax for importing and exporting code between files. This module system is widely adopted in modern front-end development, as well as in Node.js applications with newer versions.
In addition to Android app development, Java is also used for building desktop applications. JavaFX is a framework that allows developers to create rich and interactive graphical user interfaces for applications running on various platforms, including Windows, macOS, and Linux.
UMD is a module format that aims to provide compatibility between CommonJS, AMD, and global variable definitions. This flexibility allows developers to create modules that work across various environments, making it a suitable choice for libraries intended for both client-side and server-side use.
JavaScript bundlers are tools that simplify the management of modular code by combining multiple files and dependencies into a single, optimized file or a set of files. They enhance the development workflow, improve performance, and address issues related to the loading and execution of code. Let's explore some prominent JavaScript bundlers and their key features.
Webpack is a widely used JavaScript bundler that excels in handling a variety of assets beyond JavaScript, such as CSS, images, and fonts. It employs a powerful module system that supports both CommonJS and ES6 modules. Webpack analyzes the dependencies of the application and generates a bundled output accordingly.
Parcel is a zero-config bundler that simplifies the setup process for developers. It supports multiple asset types out of the box and automatically analyzes the project structure to determine the dependencies. Parcel is known for its fast build times and ease of use.
Rollup is a JavaScript bundler that focuses on creating smaller and more efficient bundles. It is particularly suitable for libraries and packages. Rollup supports ES6 modules and tree-shaking, a technique that eliminates dead code, resulting in more compact bundles.
Browserify is a bundler that allows developers to use Node.js-style modules in the browser. It recursively analyzes the project's dependencies and bundles them into a single file. Browserify is known for its simplicity and compatibility with the CommonJS module system.
JavaScript task runners are tools that automate repetitive tasks in the development workflow, making it more efficient and error-free. They are particularly useful for tasks such as code compilation, minification, testing, and more. Let's explore some prominent JavaScript task runners and their key features.
Grunt is a widely used task runner that focuses on simplicity and configurability. It uses a configuration file (usually named Gruntfile.js) to define tasks and their configurations. Grunt has a large ecosystem of plugins, allowing developers to easily extend its functionality.
Gulp is a task runner that leverages a code-over-configuration approach. It uses a gulpfile.js to define tasks as JavaScript functions, making it more flexible and expressive. Gulp promotes the use of streams, which allows for efficient manipulation of files.
While not a dedicated task runner, npm (Node Package Manager) scripts can be utilized as a lightweight alternative for running tasks. Developers can define custom scripts in the package.json file, and npm will execute them with simple commands.
For simple projects or specific use cases, developers might opt to use shell commands directly for task automation. This approach can be straightforward and requires minimal configuration.
In addition to the information laid out above, there are a handful of important terms you should also know:
Want to learn more about JavaScript? Additional information can be found in the blog posts and books listed below.
How to Parallelize Tasks in JavaScript
Learn more computing from our official Learning Center.
And to continue learning even more about JavaScript, sign up for our weekly blog recap here