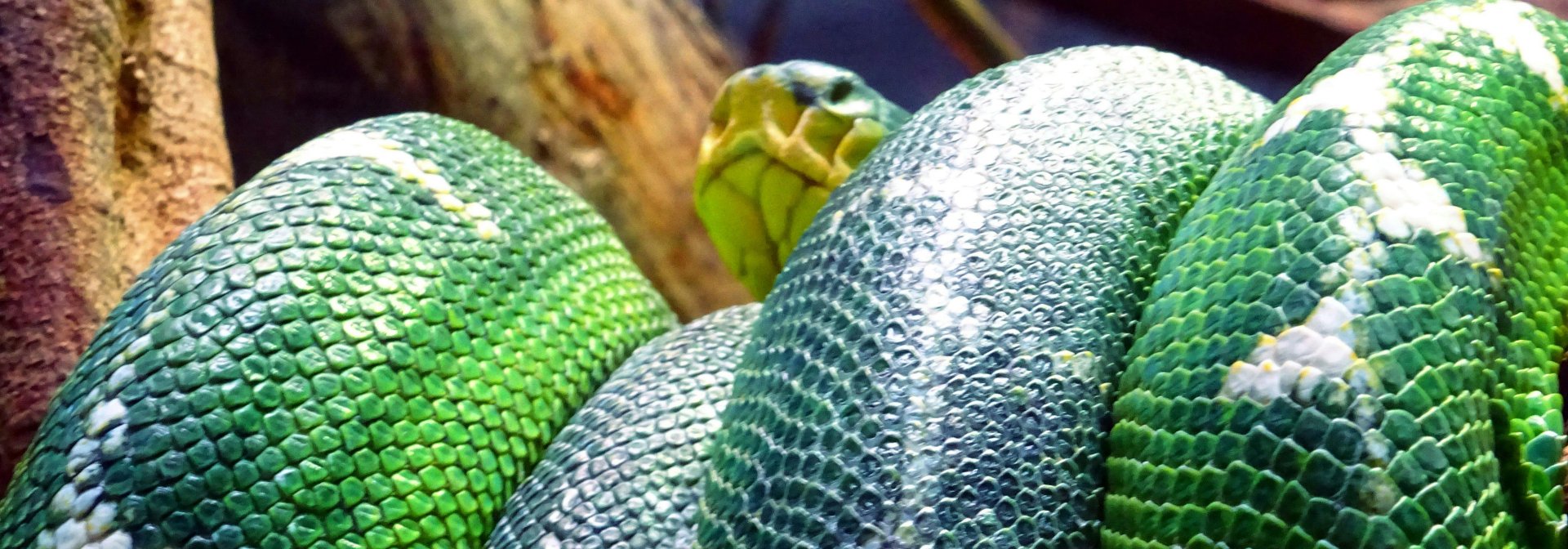
Python, often dubbed the "Swiss Army Knife" of programming languages, has emerged as one of the most versatile and widely-used languages in the world of computer science and software development. It boasts a wide range of applications, from web development and data analysis to scientific research and artificial intelligence.
Python is a high-level, interpreted programming language known for its simplicity and readability. Created by Guido van Rossum in the late 1980s, Python was designed with a focus on code readability, making it an excellent choice for beginners and experienced developers alike. Here are some essential aspects of Python.
Python's syntax is renowned for its simplicity and clarity. Its use of indentation and whitespace to define code blocks gives it a distinctive appearance and promotes consistent coding style.
The absence of curly braces and semicolons contributes to Python's clean and readable code.
Python is an interpreted language, meaning that the code is executed line by line by an interpreter without the need for compilation. This leads to a shorter development cycle and simplifies debugging.
Python is dynamically typed, allowing variables to change data types as needed. This flexibility simplifies coding but may require careful attention to variable types in larger projects.
Python includes a comprehensive standard library with modules that simplify a wide range of tasks, such as file handling, data compression, and networking.
Python Virtual Environments are crucial for managing project dependencies and isolating different projects from each other. Virtual environments help create isolated environments for Python projects, preventing conflicts between package versions. The built-in venv module or third-party tools like virtualenv are commonly used for creating virtual environments.
Creating a virtual environment in Python is quick and straightforward using tools like venv or virtualenv. With a few simple command-line instructions, developers can establish isolated spaces for projects, making dependency management efficient and preventing conflicts between projects.
Once a virtual environment is activated, you can use tools like pip to install project-specific dependencies. This ensures that each project has its own set of packages without affecting the system-wide Python installation.
Python's versatility and ease of use have made it a staple in various engineering disciplines. Whether you're designing software for control systems, simulating physical phenomena, or analyzing engineering data, Python provides the tools and libraries needed for these tasks.
Python is commonly used in control systems engineering. Libraries like control and scipy.signal offer tools for modeling, simulating, and controlling dynamic systems. Engineers can design controllers and analyze system responses with ease.
Python's libraries, such as NumPy and SciPy, provide extensive capabilities for numerical simulations and analysis. Engineers can model physical phenomena, perform finite element analysis (FEA), and solve complex equations using these libraries.
Python's data analysis and visualization libraries, including Pandas and Matplotlib, empower engineers to make sense of vast datasets and communicate their findings effectively. Whether it's analyzing sensor data or presenting simulation results, Python excels in this domain.
Python's role in scripting is undeniable. It has become a go-to language for automation, web scraping, and task scheduling due to its simplicity and cross-platform compatibility. Scripting is an essential use case for Python in various industries.
Python is widely used for automating repetitive tasks. Whether it's processing files, managing system configurations, or handling data, Python scripts can save valuable time and reduce the risk of errors.
Python's rich ecosystem of web scraping libraries, including BeautifulSoup and Scrapy, enables developers to extract data from websites for various purposes, from market research to content aggregation.
Python is ideal for creating scheduled tasks or batch processes. The schedule library, for instance, allows you to automate jobs at specified times or intervals. This is useful for performing backups, sending notifications, or running data processing tasks.
Python's libraries for data science, including NumPy, Pandas, Matplotlib, and scikit-learn, have made it the go-to language for data analysis, machine learning, and artificial intelligence. These libraries provide the tools needed to process, analyze, and derive insights from data.
Pandas is a powerful library for data manipulation and analysis. It simplifies data cleaning, transformation, and exploration.
Matplotlib is a versatile library for creating visualizations, including line charts, bar charts, and heatmaps.
Python's scikit-learn library provides a wide range of tools for machine learning, including classification, regression, clustering, and dimensionality reduction.
Python is not limited to scientific and data-related applications; it is also widely used in web development. Python web frameworks, such as Django and Flask, provide developers with the tools to create web applications, RESTful APIs, and dynamic websites.
Django is a high-level Python web framework known for its simplicity and speed of development. It follows the Model-View-Controller (MVC) architectural pattern, making it easy to structure web applications. With built-in features like authentication, admin panels, and database support, Django is an excellent choice for robust web applications.
Flask is a lightweight microframework for Python. It provides the essentials for building web applications without imposing strict architectural patterns. This flexibility makes Flask an excellent choice for small to medium-sized projects.
Pyramid is a full-featured web framework that is known for its flexibility and scalability. It allows developers to choose the right tools for their project, providing a balance between simplicity and complexity. Pyramid follows the concept of "pay only for what you use," allowing developers to add components as needed.
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It is designed to be easy to use and to provide automatic interactive documentation. FastAPI is built on top of Starlette and Pydantic.
Bottle is a simple and lightweight microframework that is distributed as a single-file module. It is easy to use and well-suited for small projects or applications where simplicity is a priority.
CherryPy is an object-oriented web framework that allows developers to build web applications in a similar way to writing Python programs. It is known for its simplicity and can be a good choice for small to medium-sized projects.
Python's web development ecosystem also includes libraries and tools for working with databases, handling user authentication, and implementing RESTful APIs.
Python's role in artificial intelligence (AI) and machine learning (ML) has skyrocketed in recent years. It serves as the language of choice for developing and deploying AI applications, training ML models, and conducting cutting-edge research. Python's rich ecosystem offers numerous libraries and frameworks for AI and ML.
TensorFlow and PyTorch are two of the most popular libraries for deep learning and neural network development. They allow researchers and developers to design complex models and train them on large datasets.
Python libraries like NLTK and spaCy are widely used for NLP tasks, including text preprocessing, sentiment analysis, and named entity recognition.
OpenAI Gym is a popular library for developing and testing reinforcement learning algorithms. It provides a wide range of environments and challenges for training intelligent agents.
Python's accessibility and extensive libraries have made it a preferred choice in education and scientific research. It is used for teaching programming, conducting experiments, and performing simulations in various scientific domains.
Python's simplicity and readability make it an excellent choice for teaching programming to beginners. Educational platforms and institutions often use Python to introduce students to coding concepts.
Python code for a simple "Hello, World!" program provides a gentle introduction to programming for newcomers.
Python is heavily used in scientific research, enabling scientists and researchers to analyze data, simulate experiments, and visualize results. Libraries like SciPy, NumPy, and Matplotlib are vital for conducting experiments and representing findings.
Python can be applied to research areas such as physics, biology, chemistry, astronomy, and climate modeling.
Python is a powerful language for developing games, thanks to libraries like Pygame and Godot Engine. These libraries simplify the process of creating 2D and 3D games, making game development accessible to a wide range of developers.
Pygame is a set of Python modules designed for writing games. It provides functions for drawing graphics, handling user input, and managing game events. With Pygame, developers can create 2D games for various platforms.
Godot Engine is a game engine that uses its scripting language, GDScript, which is similar to Python. It is suitable for both 2D and 3D game development. Godot Engine provides a visual editor for designing game levels and scenes.
Python's versatility extends to the field of cybersecurity. Security experts and penetration testers often use Python to develop tools and scripts for testing and securing systems.
Python is a preferred language for writing penetration testing scripts and tools. The Metasploit framework, along with libraries like requests, paramiko, and nmap, allows security professionals to assess the security of networks and systems.
Do note that penetration testing and ethical hacking should only be performed with proper authorization.
Python is also valuable in automating security tasks and incident response. Scripts can be written to monitor system logs, detect anomalies, and trigger responses when potential security threats are identified.
Python is widely used in the finance industry for tasks such as algorithmic trading, risk analysis, and portfolio management. Libraries like pandas, numpy, and quantlib are employed for data analysis and modeling.
Python is the preferred language for algorithmic trading due to its vast ecosystem of libraries. Traders and financial institutions use Python to develop trading strategies, analyze market data, and execute trades automatically.
Python is instrumental in risk analysis and portfolio management. Libraries like pyfolio and cvxpy enable investors and fund managers to assess portfolio risk, optimize asset allocation, and monitor portfolio performance.
Python is widely used for testing and debugging applications, thanks to its rich set of testing frameworks and debugging tools.
Python's built-in unittest module provides a framework for writing and running unit tests.
Python's built-in debugger, pdb, allows developers to interactively debug their code. Inserting the following line in the script sets a breakpoint.
Python is a versatile language for Internet of Things (IoT) development, offering simplicity and a wide range of libraries for IoT applications.
The MQTT protocol is commonly used for communication in IoT projects. The paho-mqtt library facilitates MQTT communication in Python.
Python is extensively used in cloud computing for automation, infrastructure management, and developing cloud-native applications.
Terraform, a popular Infrastructure as Code (IaC) tool, allows users to define and provision infrastructure using a declarative configuration language.
Python is a go-to language for Natural Language Processing (NLP) due to its extensive libraries and frameworks for text analysis.
The Natural Language Toolkit (NLTK) provides tools for working with human language data. Tokenizing text into words is a common NLP task.
In addition to NLTK, another popular NLP library called spaCy is also used often for this purpose.
Python is widely used in image processing and computer vision applications, leveraging libraries like OpenCV and scikit-image.
OpenCV is a powerful library for computer vision tasks.
In addition to the information laid out above, there are a handful of important terms you should also know:
Want to learn more about Python? Additional information can be found in the blog posts, books, and videos listed below.
Learn more computing from our official Learning Center.
And to continue learning even more about Python, sign up for our weekly blog recap here: